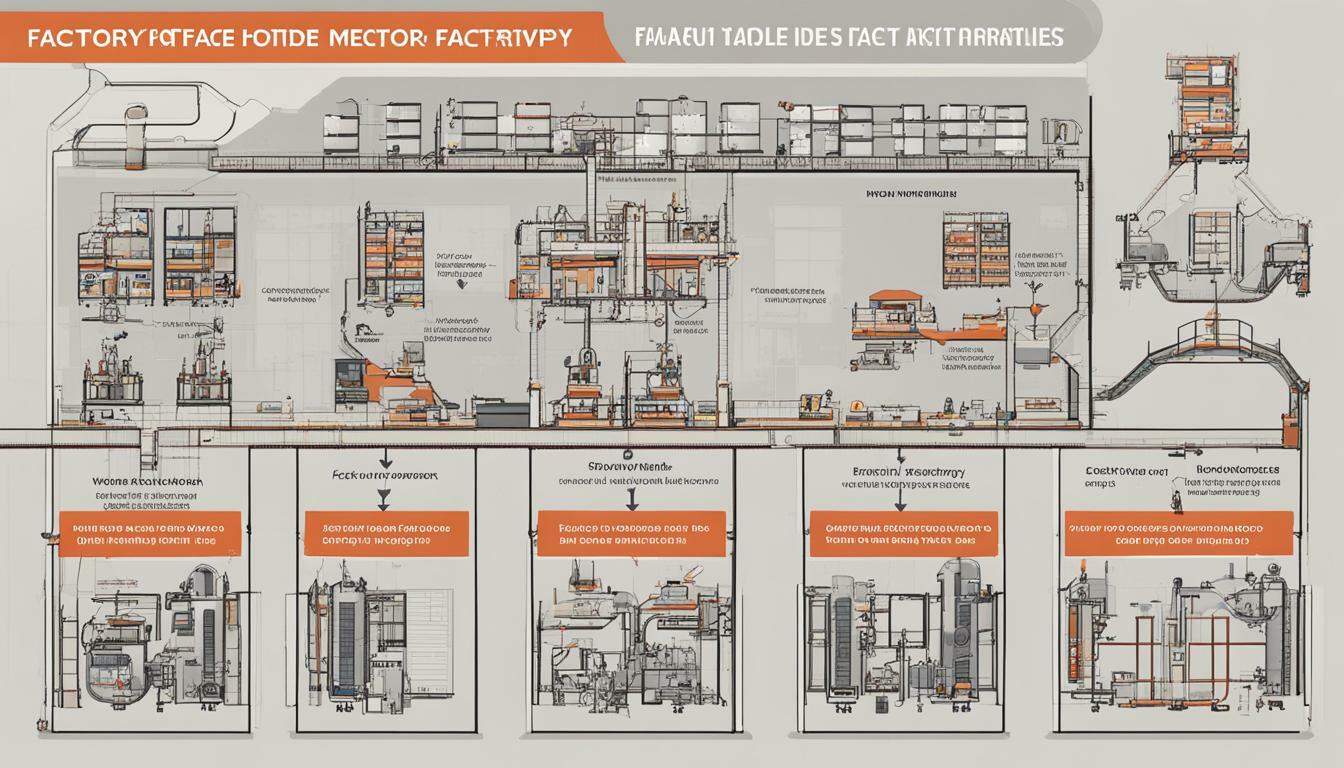
In software development, creating objects is a repetitive process. Developers use design patterns to manage this process efficiently, ensuring that code is flexible, maintainable, and reusable. Factory patterns are a set of creation patterns that simplify object creation and reduce coupling between objects.
In this article, we will compare and contrast two popular factory patterns: The Factory Method and Abstract Factory. We will explore their implementation, purpose, and use cases to help developers choose the right pattern for their projects.
Key Takeaways:
- Factory patterns simplify object creation and reduce coupling between objects
- The Factory Method and Abstract Factory are two popular factory patterns
- Understanding these patterns can improve software design and development
- Choosing the right pattern depends on the specific requirements of a project
Understanding Creation Patterns in Software Design
Software design patterns are essential for maintaining code flexibility and reusability. Creation patterns, one of the categories of design patterns, help in object creation by defining the best practices and methodologies for it. These patterns describe the process of object creation and help in managing the dependencies between objects.
Design patterns for object creation can be divided into two categories – class-creation patterns and object-creation patterns. The former is concerned with class instantiation, while the latter deals with object instantiation. Creation patterns like the Factory Method and Abstract Factory fall under object-creation patterns.
The Factory pattern provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. It encapsulates object creation logic and decouples it from the rest of the code, making it easier to maintain and test. In contrast, the Abstract Factory pattern provides an interface for creating families of related objects. It not only solves the problem of creating objects, but also manages how those objects interact.
The advantages of using creation patterns in software design are numerous. Besides reducing code complexity and making code more modular, they enable better code reusability and extensibility. By making design decisions based on patterns, developers can save time and create more robust and flexible code.
The Factory Method Pattern in Software Development
The factory pattern is an object-oriented design pattern that helps to create objects in an organized way. The Factory Method Pattern is a creational pattern that simplifies object creation by encapsulating object creation logic and providing a way for subclasses to handle object instantiation. The Factory Method pattern is used in creating a new object, but it delegates the implementation of creating that object to the subclasses.
The Factory Method pattern is essential in software development because it helps to achieve a loosely coupled and extensible system. It does this by providing a framework for object creation, which makes it easy to add new types of objects to the system. When implemented correctly, changes to the objects created by the Factory Method pattern do not affect the client code.
The Factory Method pattern is useful in various software development scenarios. It can be used when the creation of an object is complex, when multiple objects need to be created, and when the creation of the objects needs to be done in a certain order. The Factory Method pattern is also useful when the creation of objects needs to be done dynamically at runtime.
In conclusion, the Factory Pattern and Factory Method in software development provide a way to create objects in a structured and organized way. The benefits in terms of flexibility, maintainability, and extensibility make it an essential pattern in the toolbox of any software developer.
The Abstract Factory Pattern: A Deeper Look
In software development, the Abstract Factory pattern is an extension of the Factory Method pattern. It allows the creation of families of related objects, making it an ideal choice when dealing with multiple object variations. The Abstract Factory pattern is used to provide a way for creating objects with varying behavior, while keeping the object creation logic hidden from the clients.
The Abstract Factory pattern consists of a set of factory methods that are used to create a family of products. Each factory method corresponds to a different product and encapsulates the logic required for creating that specific product. The client code is then written to use the appropriate factory method based on the context, thus providing a way for creating the required set of objects in a seamless manner.
One of the key benefits of the Abstract Factory pattern is that it provides an interface for creating families of related objects. This allows for easy swapping of families during runtime, providing a high degree of flexibility to the design. Additionally, the Abstract Factory pattern can be combined with other design patterns like the Singleton and Prototype patterns to provide even more powerful solutions.
There are several use cases where the Abstract Factory pattern can be applied effectively. For example, in a game development scenario, the Abstract Factory pattern can be used to create different types of enemies, weapons, and power-ups. In a GUI application, the Abstract Factory pattern can be used to create different types of buttons, menus, and text boxes.
In conclusion, the Abstract Factory pattern is a powerful creation pattern that allows for the creation of families of related objects. It provides a high degree of flexibility to the design, and can be combined with other patterns to create even more robust solutions. When dealing with multiple object variations, the Abstract Factory pattern is an ideal choice and should be considered in software design.
The Factory Method vs. Abstract Factory: A Comparison
In software design, choosing the right creation pattern is crucial for maintaining code flexibility and reusability. The Factory Method and Abstract Factory patterns are two popular creation patterns that serve similar yet distinct purposes.
The Factory Method Pattern
The Factory Method pattern encapsulates object creation logic and provides a way for subclasses to handle object instantiation. It promotes loose coupling by allowing the client code to work with instances of concrete classes through a common interface. The Factory Method is suitable for scenarios where there are multiple concrete classes that implement the same interface, and the concrete class to be used is determined at runtime.
The Abstract Factory Pattern
The Abstract Factory pattern extends the Factory Method pattern and allows the creation of families of related objects. It provides an interface for creating families of related or dependent objects without specifying their concrete classes. The Abstract Factory is suitable for scenarios where an application needs to work with multiple families of related objects that share a common theme.
Comparison of Factory Method and Abstract Factory
Factor | Factory Method | Abstract Factory |
---|---|---|
Purpose | Encapsulates object creation logic and provides a way for subclasses to handle object instantiation. | Allows the creation of families of related objects. |
Implementation | Uses a method to create and return objects. | Uses a factory class to create and return families of related objects. |
Use Cases | Multiple concrete classes that implement the same interface, and the concrete class to be used is determined at runtime. | Multiple families of related objects that share a common theme. |
Both patterns offer benefits in terms of code flexibility and reusability. However, the Factory Method is more suitable for scenarios where there are multiple concrete classes that implement the same interface, and the concrete class to be used is determined at runtime. On the other hand, the Abstract Factory is more suitable for scenarios where an application needs to work with multiple families of related objects that share a common theme.
Benefits and Use Cases of Creation Patterns
Understanding creation patterns in software design has numerous benefits. By using these patterns, developers can ensure code flexibility, reusability, and maintainability. Creation patterns such as the Factory Method and Abstract Factory can have impactful use cases in creating and managing objects.
For instance, the Factory Method pattern encapsulates object creation logic, making it more manageable and structured. This helps in creating a consistent and modular codebase that can be scaled as a project grows. On the other hand, the Abstract Factory pattern allows the creation of related families of objects with varying behaviors. This becomes especially useful in larger projects where multiple object creation and management is required.
One real-world use case of the Factory Method pattern is in the creation of database connections. By encapsulating the database connection logic, developers can write cleaner and more maintainable code that can be used in multiple parts of the application. Similarly, the Abstract Factory pattern can be applied in building GUI widgets, where related widgets such as buttons, labels, and checkboxes need to be created with varying appearances and behaviors.
It is important to choose the right creation pattern based on the specific requirements of a software project. By doing so, developers can enhance the project’s structure, scalability and maintainability in the long run.
Conclusion
In conclusion, understanding creation patterns, such as the Factory Method and Abstract Factory, is crucial for software developers. These patterns help maintain code flexibility and reusability, while encapsulating object creation logic.
By deep diving into creation patterns, developers can choose the right pattern for their specific software project needs. The Factory Method pattern is useful for instantiating a single type of object, while the Abstract Factory pattern can create families of related objects with varying behavior.
Ultimately, choosing the appropriate creation pattern can improve software design and development. So, the next time you embark on a software development project, remember to deep dive into creation patterns.
FAQ
Q: What are creation patterns in software design?
A: Creation patterns, also known as design patterns for object creation, are techniques used in software design to facilitate the creation of objects in a flexible and reusable manner. These patterns help developers maintain code organization, improve code structure, and enable easy modification and extension of object creation logic.
Q: What is the Factory Method pattern?
A: The Factory Method pattern is a design pattern that provides a way for subclasses to handle the instantiation of objects. It encapsulates the object creation logic within a factory method, allowing subclasses to determine which concrete class should be instantiated. This pattern promotes loose coupling and flexibility in object creation and is commonly used in scenarios where a class cannot anticipate the exact types of objects it needs to create.
Q: What is the Abstract Factory pattern?
A: The Abstract Factory pattern is an extension of the Factory Method pattern. It allows the creation of families of related objects without specifying their concrete classes. Instead of having individual factory methods for each type of object, an abstract factory interface is used to define a set of related object creation methods. Concrete subclasses of the abstract factory implement these methods to create objects with varying behaviors.
Q: What are the similarities and differences between the Factory Method and Abstract Factory patterns?
A: Both the Factory Method and Abstract Factory patterns are used to abstract the process of object creation. They promote loose coupling and flexibility in object instantiation. The key difference is that the Factory Method pattern focuses on individual object creation, allowing subclasses to handle the creation of specific objects. On the other hand, the Abstract Factory pattern deals with the creation of families of related objects, abstracting the creation of multiple objects with varying behaviors.
Q: What are the benefits of understanding creation patterns?
A: Understanding creation patterns helps improve software design by promoting code organization, flexibility, and reusability. These patterns enable developers to easily modify and extend object creation logic without impacting the rest of the codebase. By choosing the appropriate creation pattern, developers can create maintainable and scalable software solutions.
Q: In what use cases can the Factory Method and Abstract Factory patterns be applied?
A: The Factory Method pattern is effective in scenarios where a class needs to delegate the creation of objects to its subclasses. It allows for easy extension and customization of object creation. The Abstract Factory pattern is suitable when a system needs to create families of related objects that share common behavior. It enables the creation of objects with varying behaviors and ensures the consistency of the entire family of objects.
Q: What are the key takeaways from this article?
A: In this article, we explored and compared the Factory Method and Abstract Factory patterns. We learned about creation patterns in software design and their benefits. The Factory Method pattern encapsulates object creation logic, while the Abstract Factory pattern extends it to create families of related objects. Understanding these patterns can greatly improve software design and enable flexibility in object creation.